《iOS多线程简介》中提到:GCD中有2个核心概念:1、任务(执行什么操作)2、队列(用来存放任务)
那么多线程GCD的基本使用有哪些呢?
可以分以下多种情况:
1、异步函数 + 并发队列
/**
* 异步函数 + 并发队列:可以同时开启多条线程
*/
- (void)asyncConcurrent
{
// 1.创建一个并发队列
// dispatch_queue_create(const char *label, dispatch_queue_attr_t attr);
// label : 相当于队列的名字
// dispatch_queue_t queue = dispatch_queue_create("com.kyle.queue", DISPATCH_QUEUE_CONCURRENT);
// 1.获得全局的并发队列
dispatch_queue_t queue = dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0);
// 2.将任务加入队列
dispatch_async(queue, ^{
for (NSInteger i = 0; i<3; i++) {
NSLog(@"this is first %@",[NSThread currentThread]);
}
});
dispatch_async(queue, ^{
for (NSInteger i = 0; i<3; i++) {
NSLog(@"this is second %@",[NSThread currentThread]);
}
});
dispatch_async(queue, ^{
for (NSInteger i = 0; i<3; i++) {
NSLog(@"this is third %@",[NSThread currentThread]);
}
});
}
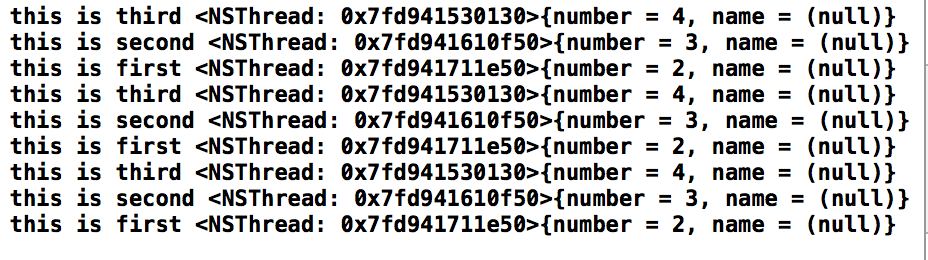
2、同步函数 + 并发队列
/**
* 同步函数 + 并发队列:不会开启新的线程
*/
- (void)syncConcurrent
{
// 1.获得全局的并发队列
dispatch_queue_t queue = dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0);
// 2.将任务加入队列
dispatch_sync(queue, ^{
NSLog(@"this is first %@",[NSThread currentThread]);
});
dispatch_sync(queue, ^{
NSLog(@"this is second %@",[NSThread currentThread]);
});
dispatch_sync(queue, ^{
NSLog(@"this is third %@",[NSThread currentThread]);
});
}

3、异步函数 + 串行队列
/**
* 异步函数 + 串行队列:会开启新的线程,但是任务是串行的,执行完一个任务,再执行下一个任务
*/
- (void)asyncSerial
{
// 1.创建串行队列
dispatch_queue_t queue = dispatch_queue_create("com.kyle.queue", DISPATCH_QUEUE_SERIAL);
// dispatch_queue_t queue = dispatch_queue_create("com.kyle.queue", NULL);
// 2.将任务加入队列
dispatch_async(queue, ^{
NSLog(@"this is first %@",[NSThread currentThread]);
});
dispatch_async(queue, ^{
NSLog(@"this is second %@",[NSThread currentThread]);
});
dispatch_async(queue, ^{
NSLog(@"this is third %@",[NSThread currentThread]);
});
}

4、同步函数 + 串行队列
/**
* 同步函数 + 串行队列:不会开启新的线程,在当前线程执行任务。任务是串行的,执行完一个任务,再执行下一个任务
*/
- (void)syncSerial
{
// 1.创建串行队列
dispatch_queue_t queue = dispatch_queue_create("com.kyle.queue", DISPATCH_QUEUE_SERIAL);
// 2.将任务加入队列
dispatch_sync(queue, ^{
NSLog(@"this is first %@",[NSThread currentThread]);
});
dispatch_sync(queue, ^{
NSLog(@"this is second %@",[NSThread currentThread]);
});
dispatch_sync(queue, ^{
NSLog(@"this is third %@",[NSThread currentThread]);
});
}

5、异步函数 + 主队列
// * 异步函数 + 主队列:只在主线程中执行任务
- (void)asyncMain
{
// 1.获得主队列
dispatch_queue_t queue = dispatch_get_main_queue();
// 2.将任务加入队列
dispatch_async(queue, ^{
NSLog(@"this is first %@",[NSThread currentThread]);
NSLog(@"this is second %@",[NSThread currentThread]);
NSLog(@"this is third %@",[NSThread currentThread]);
});
}

6、同步函数 + 主队列
// * 同步函数 + 主队列:
- (void)syncMain
{
NSLog(@"begin");
// 1.获得主队列
dispatch_queue_t queue = dispatch_get_main_queue();
// 2.将任务加入队列
dispatch_sync(queue, ^{
NSLog(@"this is %@",[NSThread currentThread]);
});
NSLog(@"end");
}
造成“相互等待的